rueki
5. pytorch를 이용한 Linear Regression 본문
728x90
반응형
import torch
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
import torch.nn as nn
X = torch.linspace(1,50,50).reshape(-1,1) # 1부터 50까지 50 step
# 크기에 맞춰서 1열 형태로
X
'''
tensor([[ 1.],
[ 2.],
[ 3.],
[ 4.],......
'''
torch.manual_seed(71)
e = torch.randint(-8,9,(50,1), dtype=torch.float) # error 값, -8부터 9 까지 50 x 1 크기로
y = 2*X + 1 + e #함수 정의
plt.scatter(X.numpy(),y.numpy())
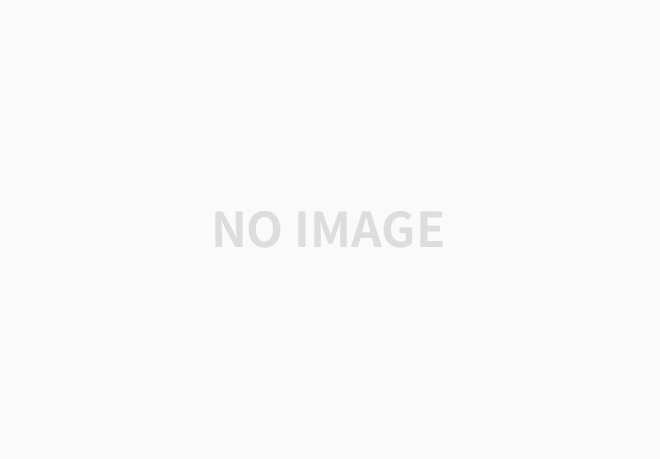
torch.manual_seed(59)
model = nn.Linear(in_features = 1, out_feautures = 1) #선형 회귀로 입력 1 출력 1개씩
print(model.weight)#가중치
print(model.bias)#편향
'''
Parameter containing:
tensor([[0.1060]], requires_grad=True)
Parameter containing:
tensor([0.9638], requires_grad=True)
'''
#모델 생성
class Model(nn.Module):
def __init__(self,in_features, out_features):
super().__init__()
self.linear = nn.Linear(in_features, out_features) # fully connected layer
def forward(self,x):
y_pred = self.linear(x)
return y_pred
torch.manual_seed(59)
model = Model(1,1)
print(model.linear.weight)
print(model.linear.bias)
'''
Parameter containing:
tensor([[0.1060]], requires_grad=True)
Parameter containing:
tensor([0.9638], requires_grad=True)
'''
# 모델 파라메터 이름과 값 출력
for name, param in model.named_parameters():
print(name,'\t',param.item())
'''
linear.weight 0.10597813129425049
linear.bias 0.9637961387634277
'''
#x값을 선언하고 모델에 적용
x = torch.tensor([2.0])
print(model.forward(x))
# tensor([1.1758], grad_fn=<AddBackward0>)
x1 = np.linspace(0.0,50.0,50)
w1 = 0.1059
b1 = 0.9637
y1 = w1 * x1 +b1
plt.scatter(X.numpy(), y.numpy())
plt.plot(x1,y1,'r')
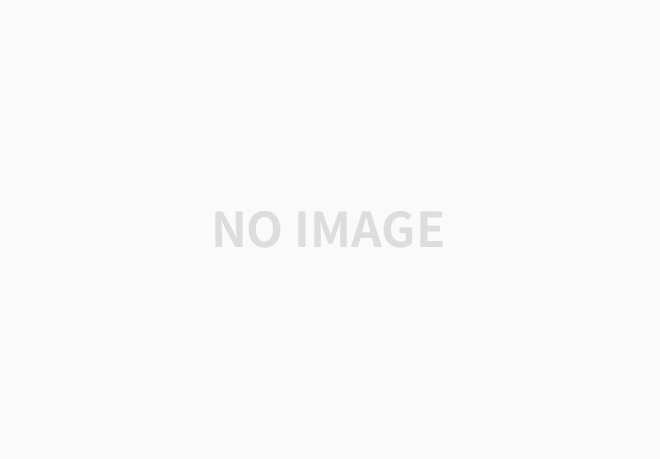
criterion = nn.MSELoss() # 손실함수 - Mean Squared Error 사용
optimizer = torch.optim.SGD(model.parameters(), lr=0.001) # 모델의 파라메터 최적화
epochs = 50
losses = []
for i in range(epochs):
i = i+1
#순전파
y_pred = model.foward(X)
#손실 계산
loss = criterion(y_pred, y)#예측값과 실제 값 차이
losses.append(loss)
print(f"epoch {i} loss : {loss.item()} weight : {model.linear.weight.item()} bias: {model.linear.bias.item()}")
#매 반복회수마다 최적화 한 값 초기화
optimizer.zero_grad()
#역전파 수행
loss.backward()
#기울기 하강 한단계 수행
optimizer.step()
plt.plot(range(epochs), losses)
plt.ylabel('MSE LOSS')
plt.xlabel('Epoch')
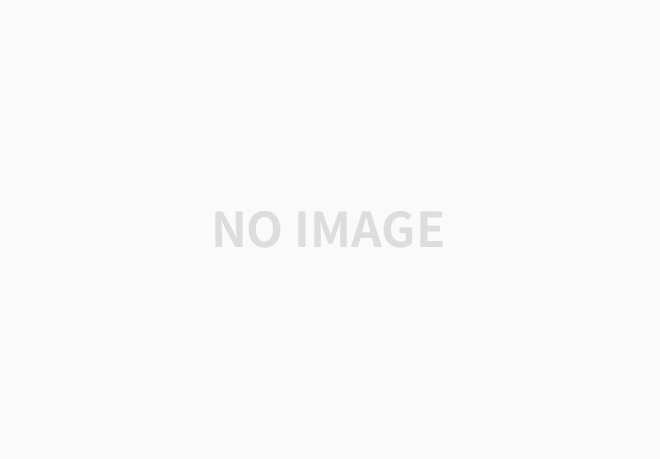
# 새 데이터 생성
x = np.linspace(0.0,50.0,50)
current_weight = model.linear.weight.item()
current_bias = model.linear.bias.item()
predicted_y = current_weight *x + current_bias
plt.scatter(X.numpy(),y.numpy())
plt.plot(x,predicted_y,'r')
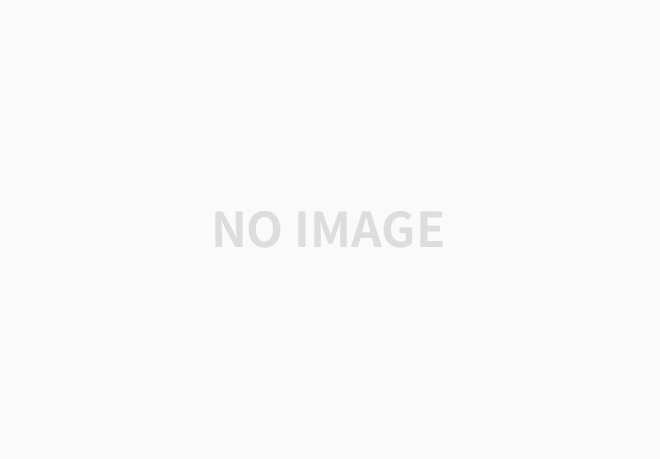
728x90
반응형
'pytorch' 카테고리의 다른 글
6. Pytorch를 이용한 MNIST 이미지 ANN Classification (5) | 2020.03.21 |
---|---|
6. Pytorch를 이용한 ANN 구현 (0) | 2020.03.07 |
4. Gradient Descent (0) | 2020.03.05 |
3. 파이토치 기본 연산 (0) | 2020.03.05 |
2. 텐서 기초2 (0) | 2020.03.04 |